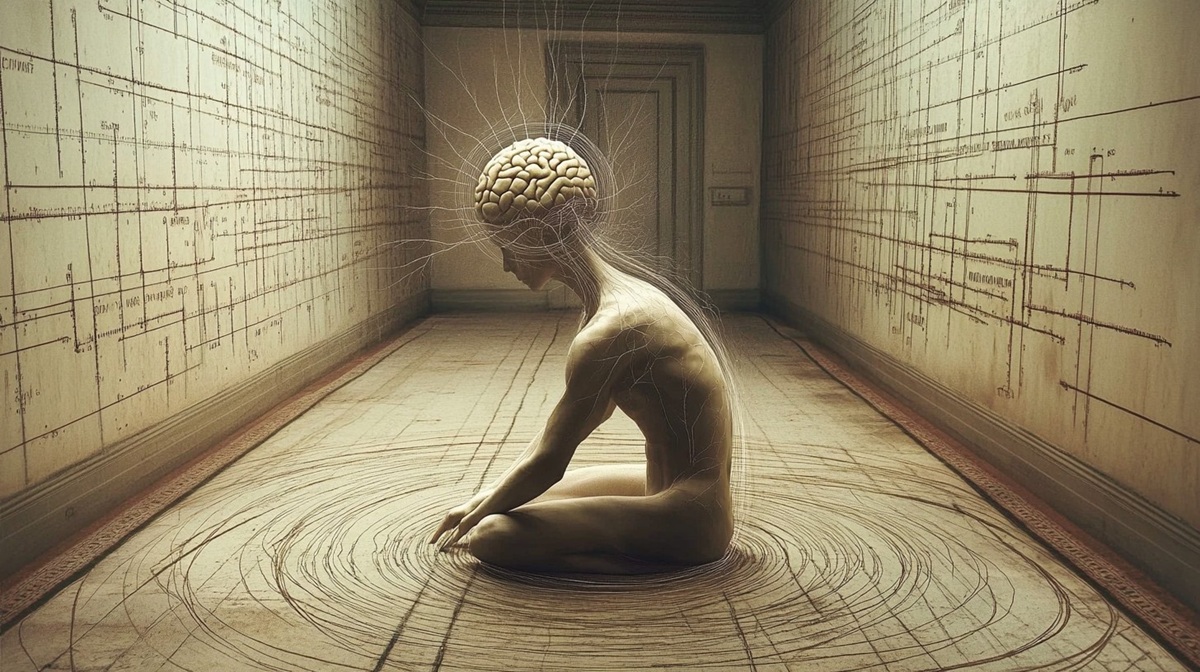
[]
Advanced LLM Prompting: Beyond Basic Instructions
A deep dive into sophisticated LLM prompting techniques, including chain-of-thought, zero-shot learning, and emergent abilities.
As Large Language Models continue to evolve, advanced prompting techniques have become crucial for unlocking their full potential. This post explores cutting-edge approaches to LLM interaction.
Prompting Paradigms
1. Basic Structure
┌────────────┐
│ Context │
└─────┬──────┘
│
┌─────▼──────┐
│ Instruction│
└─────┬──────┘
│
┌─────▼──────┐
│ Input │
└────────────┘
2. Advanced Patterns
Modern prompting incorporates:
class PromptTemplate:
def __init__(self):
self.components = {
'context': [],
'examples': [],
'constraints': [],
'instructions': []
}
def build_prompt(self):
return self.format_components(self.components)
Advanced Techniques
1. Chain-of-Thought Prompting
Question
│
┌──▼──┐
│Think│
└──┬──┘
│
┌──▼──┐
│Solve│
└──┬──┘
│
┌──▼──┐
│Check│
└──┬──┘
│
Answer
Implementation:
def chain_of_thought(question):
steps = [
"Let's approach this step by step:",
"1. First, understand the key elements",
"2. Break down the problem",
"3. Solve each component",
"4. Verify the solution"
]
return "\n".join(steps)
2. Zero-Shot Learning
Enabling models to handle new tasks:
class ZeroShotPrompt:
def __init__(self):
self.template = """
Task: {task}
Format: {format}
Constraints: {constraints}
Input: {input}
"""
def generate(self, task, format, constraints, input):
return self.template.format(
task=task,
format=format,
constraints=constraints,
input=input
)
Emergent Abilities
1. Self-Reflection
Input ───────┐
│
Analysis ────┼──► Output
│
Reflection ──┘
2. Meta-Learning
Teaching models to learn:
class MetaLearningPrompt:
def __init__(self):
self.stages = {
'observe': self.create_observation,
'analyze': self.analyze_pattern,
'apply': self.apply_learning
}
def generate_prompt(self, task):
return "\n".join(
stage(task) for stage in self.stages.values()
)
Advanced Patterns
1. Context Engineering
Sophisticated context management:
class ContextManager:
def __init__(self):
self.history = []
self.relevance_threshold = 0.8
def add_context(self, context):
self.history.append(context)
def get_relevant_context(self, query):
return [
ctx for ctx in self.history
if self.calculate_relevance(ctx, query) > self.relevance_threshold
]
2. Instruction Optimization
Clear ─────┐
│
Specific ──┼──► Instructions
│
Testable ──┘
Implementation Strategies
1. Prompt Templates
Structured prompt generation:
class AdvancedPrompt:
def __init__(self):
self.sections = {
'context': self.build_context,
'instruction': self.build_instruction,
'examples': self.build_examples,
'input': self.build_input,
'format': self.build_format
}
def generate(self, params):
return "\n\n".join(
section(params) for section in self.sections.values()
)
2. Response Formatting
┌────────────┐
│ Structure │
└─────┬──────┘
│
┌─────▼──────┐
│ Content │
└─────┬──────┘
│
┌─────▼──────┐
│ Validation │
└────────────┘
Advanced Applications
1. Code Generation
Specialized prompting for code:
class CodePrompt:
def __init__(self):
self.template = """
Language: {language}
Task: {task}
Constraints:
{constraints}
Example:
{example}
Implementation:
"""
2. Creative Tasks
Prompting for creativity:
def creative_prompt(task):
return {
'objective': task,
'constraints': [],
'inspiration': [],
'evaluation_criteria': []
}
Future Directions
1. Dynamic Prompting
Input ──────┐
│
Context ────┼──► Prompt
│
Feedback ───┘
2. Adaptive Systems
class AdaptivePrompting:
def __init__(self):
self.history = []
self.strategies = []
def adapt(self, feedback):
performance = self.analyze_feedback(feedback)
self.update_strategies(performance)
return self.generate_next_prompt()
Best Practices
1. Clarity
- Be specific and unambiguous
- Provide clear constraints
- Include success criteria
2. Structure
Setup ─────┐
│
Task ──────┼──► Prompt
│
Validation ┘
Conclusion
Advanced prompting is key to maximizing LLM capabilities. Success requires:
- Understanding model behavior
- Structured approach
- Continuous refinement
- Clear evaluation criteria
Note: These techniques represent the state of LLM prompting as of 2025. The field continues to evolve rapidly.