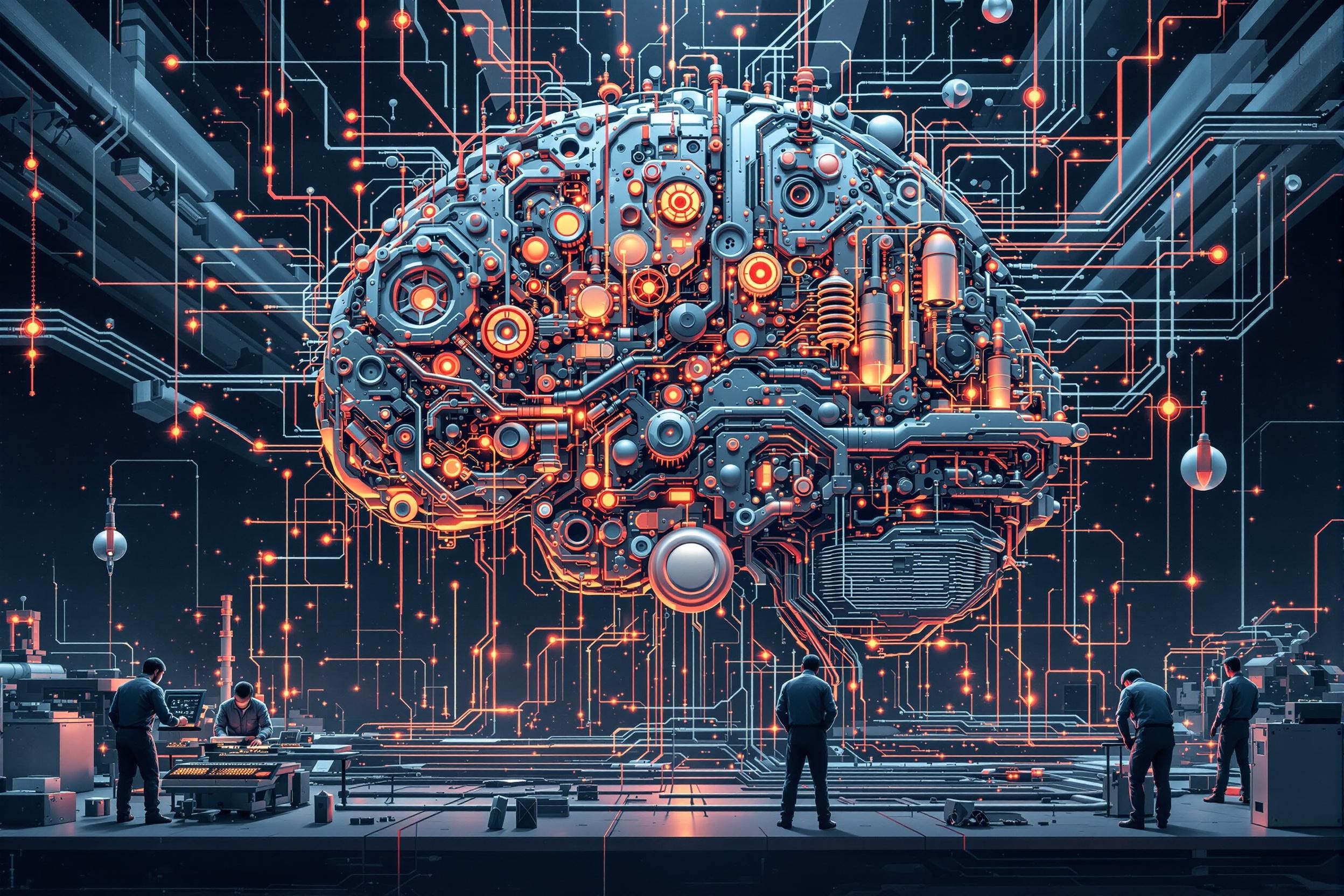
[]
Understanding LLM Scaling Laws: The Mathematics Behind AI Growth
A deep dive into the mathematical principles governing how Large Language Models scale with compute, data, and parameters.
The remarkable capabilities of modern Large Language Models (LLMs) are fundamentally governed by mathematical relationships known as scaling laws. These laws describe how model performance improves with increases in compute, data, and model size. This post explores these relationships and their implications for AI development.
The Fundamental Scaling Laws
The core scaling laws can be expressed mathematically:
import numpy as np
import matplotlib.pyplot as plt
def compute_scaling_law(N, alpha=0.7):
"""
Chinchilla-style scaling law
N: number of parameters
alpha: scaling exponent
"""
return 1.69 * (N ** alpha)
def loss_scaling(C, D, N):
"""
Loss scaling with compute, data, and parameters
C: compute in FLOPs
D: dataset size in tokens
N: number of parameters
"""
return 1.69 * (C ** -0.050) * (D ** -0.095) * (N ** -0.116)
Key Scaling Relationships
1. Compute Scaling
The relationship between model performance and computational resources follows a power law:
- Training Compute: L ∝ C^(-0.050)
- Inference Compute: Scales with model size and context length
- Memory Requirements: Generally linear with parameter count
2. Data Scaling
Data requirements scale with model size:
def optimal_dataset_size(N):
"""
Calculate optimal dataset size based on parameter count
Following Chinchilla scaling laws
"""
return 20 * N # Approximate relationship
def training_tokens_needed(model_size_params):
sizes = {
'7B': 1.4e12, # GPT-3 175B equivalent tokens
'13B': 2.6e12, # Optimal tokens for 13B model
'70B': 1.4e13, # Theoretical optimal for 70B
'175B': 3.5e13 # Full GPT-3 size
}
return sizes.get(model_size_params, 'Unknown size')
3. Parameter Efficiency
The relationship between model size and performance:
-
Parameter Counts:
- Small models (1B-10B): Limited but efficient
- Medium models (10B-100B): Sweet spot for many tasks
- Large models (100B+): Diminishing returns begin
-
Architecture Efficiency:
- Attention mechanisms scale with sequence length
- Layer counts affect depth of reasoning
- Width affects representational capacity
Modern Scaling Techniques
1. Efficient Scaling Methods
class ScalingOptimization:
def __init__(self, base_model_size):
self.base_size = base_model_size
def calculate_optimal_config(self):
"""
Calculate optimal training configuration
based on Chinchilla scaling laws
"""
params = self.base_size
return {
'dataset_size': optimal_dataset_size(params),
'training_compute': self.compute_required(params),
'batch_size': self.optimal_batch_size(params)
}
def compute_required(self, params):
"""Estimate compute required in FLOPs"""
return 6.0 * params * self.optimal_dataset_size(params)
2. Architecture Scaling
Modern approaches to scaling model architectures:
-
Mixture of Experts:
- Conditional computation paths
- Sparse activation patterns
- Reduced compute requirements
-
Attention Mechanisms:
- Linear attention variants
- Sparse attention patterns
- Efficient context handling
Practical Implications
1. Cost-Performance Trade-offs
Understanding the economics of scaling:
- Training Costs: Approximately linear with compute
- Inference Costs: Depends on deployment strategy
- Storage Requirements: Linear with parameter count
2. Optimal Training Regimes
def optimal_training_schedule(model_size, compute_budget):
"""
Calculate optimal training schedule given constraints
"""
class TrainingSchedule:
def __init__(self, steps, lr, batch_size):
self.steps = steps
self.learning_rate = lr
self.batch_size = batch_size
# Calculate based on scaling laws
steps = int(np.sqrt(compute_budget * model_size))
lr = 0.0015 * np.sqrt(batch_size)
return TrainingSchedule(steps, lr, optimal_batch_size)
Future Directions
1. Breaking Current Scaling Laws
Emerging approaches to surpass current limitations:
- Neural Architecture Search: Automated architecture optimization
- Sparse Training: Reduced parameter requirements
- Data Efficiency: Better use of available data
2. New Scaling Paradigms
class EmergingScalingLaws:
def __init__(self):
self.techniques = {
'sparse_scaling': lambda x: x * np.log(x), # Sub-linear scaling
'mixture_experts': lambda x: x * 0.7, # Reduced compute needs
'efficient_attention': lambda x: np.sqrt(x) # Sub-quadratic attention
}
def estimate_improvement(self, baseline, technique):
"""Estimate improvement over traditional scaling"""
return self.techniques[technique](baseline)
Practical Applications
1. Model Size Selection
Guidelines for choosing model size:
-
Task Requirements:
- Simple tasks: 1B-7B parameters
- Medium complexity: 7B-70B parameters
- Complex reasoning: 70B+ parameters
-
Resource Constraints:
- Training compute availability
- Inference latency requirements
- Deployment environment limitations
2. Training Strategy
Optimizing training for different scales:
def design_training_strategy(model_size, compute_budget, time_constraint):
"""
Design optimal training strategy given constraints
"""
if compute_budget < 1e20: # Small-scale training
return {
'precision': 'mixed_precision',
'parallelism': 'data_parallel',
'optimization': 'adam_with_warmup'
}
else: # Large-scale training
return {
'precision': 'bf16',
'parallelism': 'model_parallel',
'optimization': 'distributed_fused_adam'
}
Conclusion
Understanding scaling laws is crucial for efficient AI development. These mathematical relationships guide decisions about model size, training data requirements, and computational resources. As we continue to push the boundaries of AI capabilities, new scaling paradigms may emerge, but the fundamental principles of efficient scaling will remain essential for practical AI development.
The future of LLM development lies not just in scaling up, but in scaling smartly—finding ways to achieve better performance with fewer resources through architectural innovations and improved training methodologies.