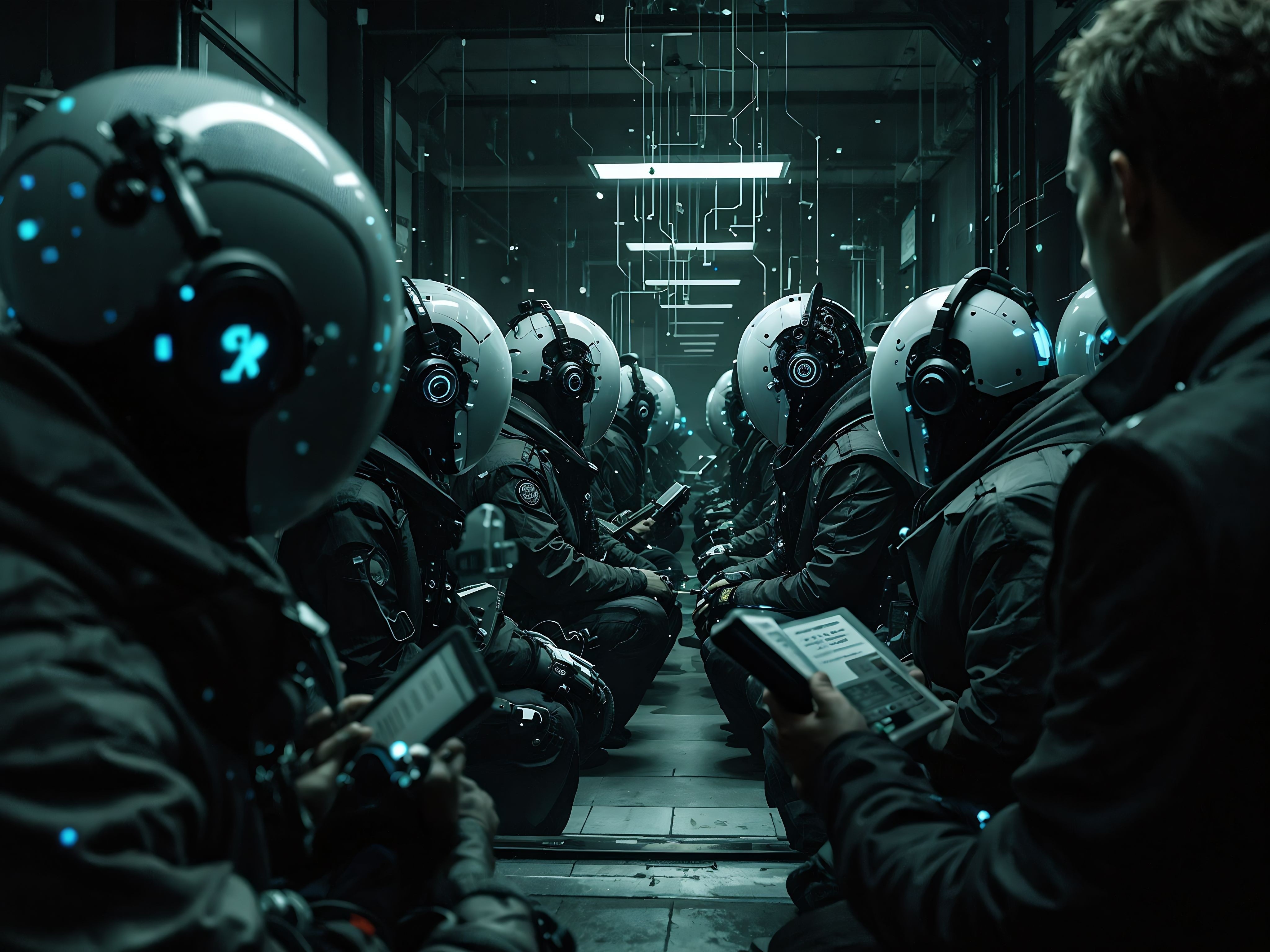
[]
The Rise of Agentic AI: Building Effective LLM Agents
A comprehensive guide to developing and deploying autonomous AI agents using Large Language Models, with best practices and implementation patterns.
The emergence of Agentic AI represents a paradigm shift in how we interact with and deploy Large Language Models (LLMs). Unlike traditional prompt-response systems, agentic AI systems can operate autonomously, make decisions, and execute complex tasks with minimal human intervention. This comprehensive guide explores the principles, best practices, and implementation patterns for building effective LLM agents.
Understanding Agentic AI
At its core, an agentic AI system possesses several key characteristics:
class AgentCapabilities:
def __init__(self):
self.autonomy = True # Independent decision-making
self.persistence = True # Maintains context and state
self.goal_oriented = True # Pursues defined objectives
self.tool_usage = True # Can use external tools/APIs
self.self_reflection = True # Can evaluate its own actions
class LLMAgent:
def __init__(self, model: str, capabilities: AgentCapabilities):
self.memory = WorkingMemory()
self.tools = ToolRegistry()
self.planning = PlanningModule()
self.reflection = ReflectionEngine()
async def execute_task(self, task: Task) -> Result:
plan = self.planning.create_plan(task)
while not task.is_complete():
next_action = plan.next_action()
result = await self.execute_action(next_action)
self.reflection.evaluate(result)
plan.adjust_if_needed(result)
Core Components of Effective Agents
1. Memory Systems
Implementing robust memory systems is crucial for agent effectiveness:
- Working Memory: Short-term context for current task
- Episodic Memory: Past experiences and outcomes
- Semantic Memory: General knowledge and facts
- Procedural Memory: Learned skills and procedures
2. Tool Usage
Agents should be equipped with a diverse toolkit:
class ToolRegistry:
def __init__(self):
self.tools = {
'file_operations': FileSystem(),
'web_access': WebClient(),
'code_execution': CodeRunner(),
'database': DatabaseClient(),
'api_calls': APIGateway()
}
def select_tool(self, task_requirements):
return self.tools[self.determine_best_tool(task_requirements)]
3. Planning and Execution
Effective planning is critical for complex task completion:
-
Task Decomposition
- Break down complex tasks into manageable subtasks
- Identify dependencies and prerequisites
- Establish success criteria
-
Action Selection
- Choose appropriate tools for each subtask
- Consider resource constraints
- Account for potential failures
-
Progress Monitoring
- Track task completion status
- Measure performance metrics
- Adjust plans based on outcomes
Best Practices for Agent Development
1. Safety and Control
class SafetyProtocols:
def __init__(self):
self.boundaries = {
'file_access': ['read_only', 'specified_directories'],
'network_access': ['allowlisted_domains', 'rate_limits'],
'system_commands': ['restricted_permissions', 'sandboxed']
}
def validate_action(self, action: Action) -> bool:
return self.check_boundaries(action) and self.verify_permissions(action)
2. Prompt Engineering
Key principles for effective agent prompts:
- Clear Role Definition: Establish agent identity and purpose
- Explicit Constraints: Define boundaries and limitations
- Success Criteria: Specify expected outcomes
- Error Handling: Include recovery procedures
3. Context Management
class ContextManager:
def __init__(self):
self.current_context = {}
self.context_history = []
self.max_context_length = 4096
def update_context(self, new_information):
self.prune_if_needed()
self.current_context.update(new_information)
self.context_history.append(new_information)
Advanced Agent Patterns
1. Multi-Agent Systems
Implementing collaborative agent networks:
- Role Specialisation: Agents with specific expertise
- Communication Protocols: Inter-agent messaging
- Conflict Resolution: Managing competing objectives
- Resource Sharing: Efficient resource allocation
2. Learning and Adaptation
class AdaptiveBehaviour:
def __init__(self):
self.learning_rate = 0.01
self.experience_buffer = []
def learn_from_experience(self, experience):
self.experience_buffer.append(experience)
self.update_behaviour_model()
self.adjust_strategies()
3. Error Recovery
Robust error handling strategies:
-
Graceful Degradation
- Fallback options for failed actions
- Alternative approach selection
- Safe state maintenance
-
Self-Repair
- Error diagnosis capabilities
- Automatic recovery procedures
- State restoration mechanisms
Implementation Guidelines
1. Development Workflow
Follow these steps for agent development:
-
Requirement Analysis
- Define agent objectives
- Identify required capabilities
- Specify constraints
-
Architecture Design
- Choose component structure
- Plan interaction patterns
- Design safety mechanisms
-
Implementation
- Build core components
- Integrate safety measures
- Implement monitoring
-
Testing and Validation
- Verify behaviour
- Test edge cases
- Validate safety measures
2. Deployment Considerations
Key factors for production deployment:
- Scaling Strategy: Handle multiple instances
- Monitoring Setup: Track performance metrics
- Resource Management: Optimise resource usage
- Update Procedures: Maintain and upgrade agents
Future Directions
The field of agentic AI continues to evolve:
-
Enhanced Autonomy
- More sophisticated decision-making
- Improved self-reflection capabilities
- Better learning from experience
-
Improved Collaboration
- More effective multi-agent systems
- Better human-agent interaction
- Enhanced communication protocols
-
Advanced Safety
- More robust control mechanisms
- Better alignment with human values
- Improved error prevention
Conclusion
Building effective LLM agents requires careful consideration of multiple factors, from core architecture to safety protocols. By following these best practices and implementation patterns, developers can create robust, reliable, and useful agentic AI systems that can safely and effectively assist in complex tasks.
Remember that the field of agentic AI is rapidly evolving, and staying current with new developments, safety measures, and best practices is crucial for building successful agent systems.
Related Posts
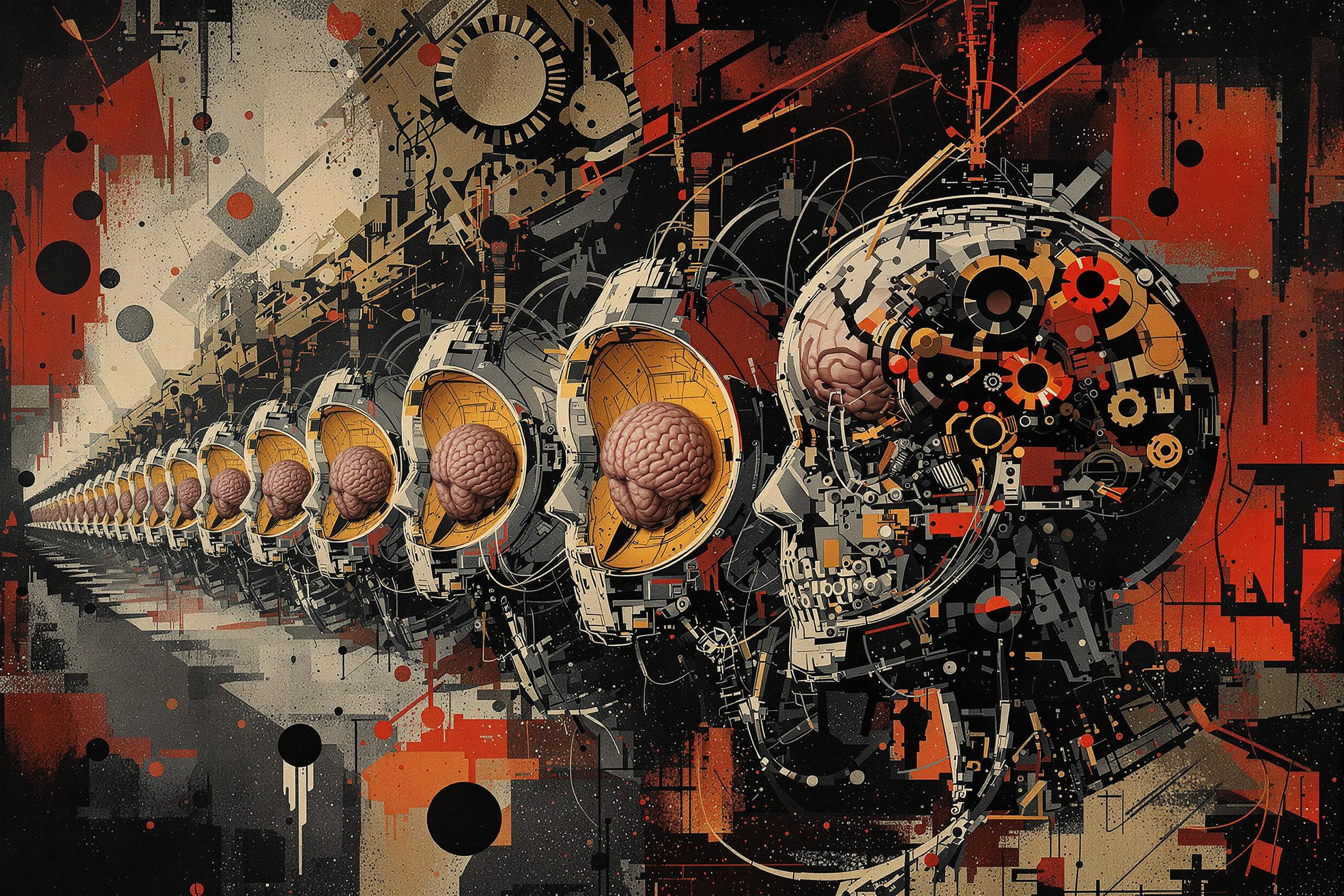
From Neurons to Neural Networks: How Neuroscience Shapes Modern AI
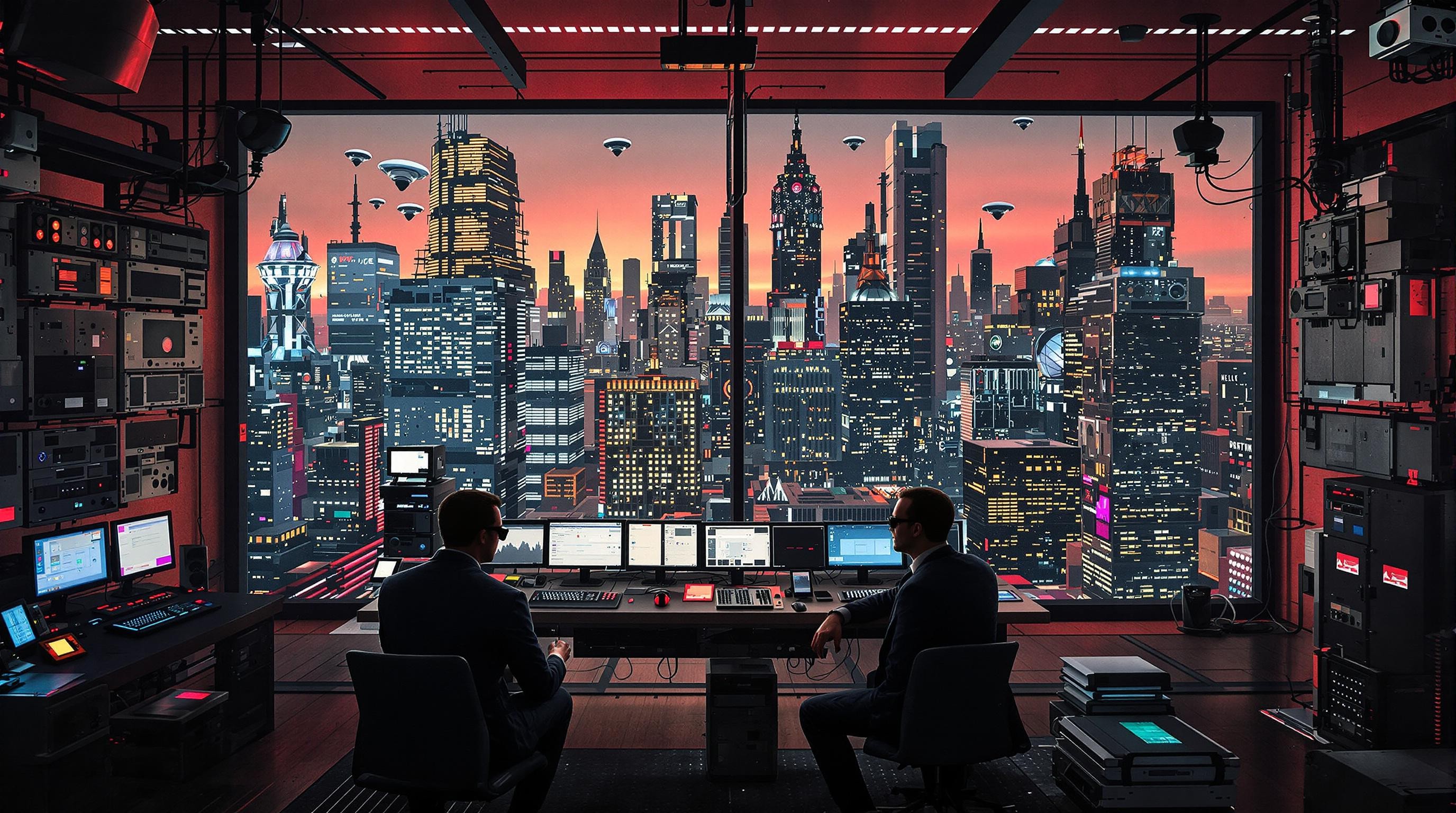
The Dark Side of Intelligence: Deceptive Behaviours in LLMs
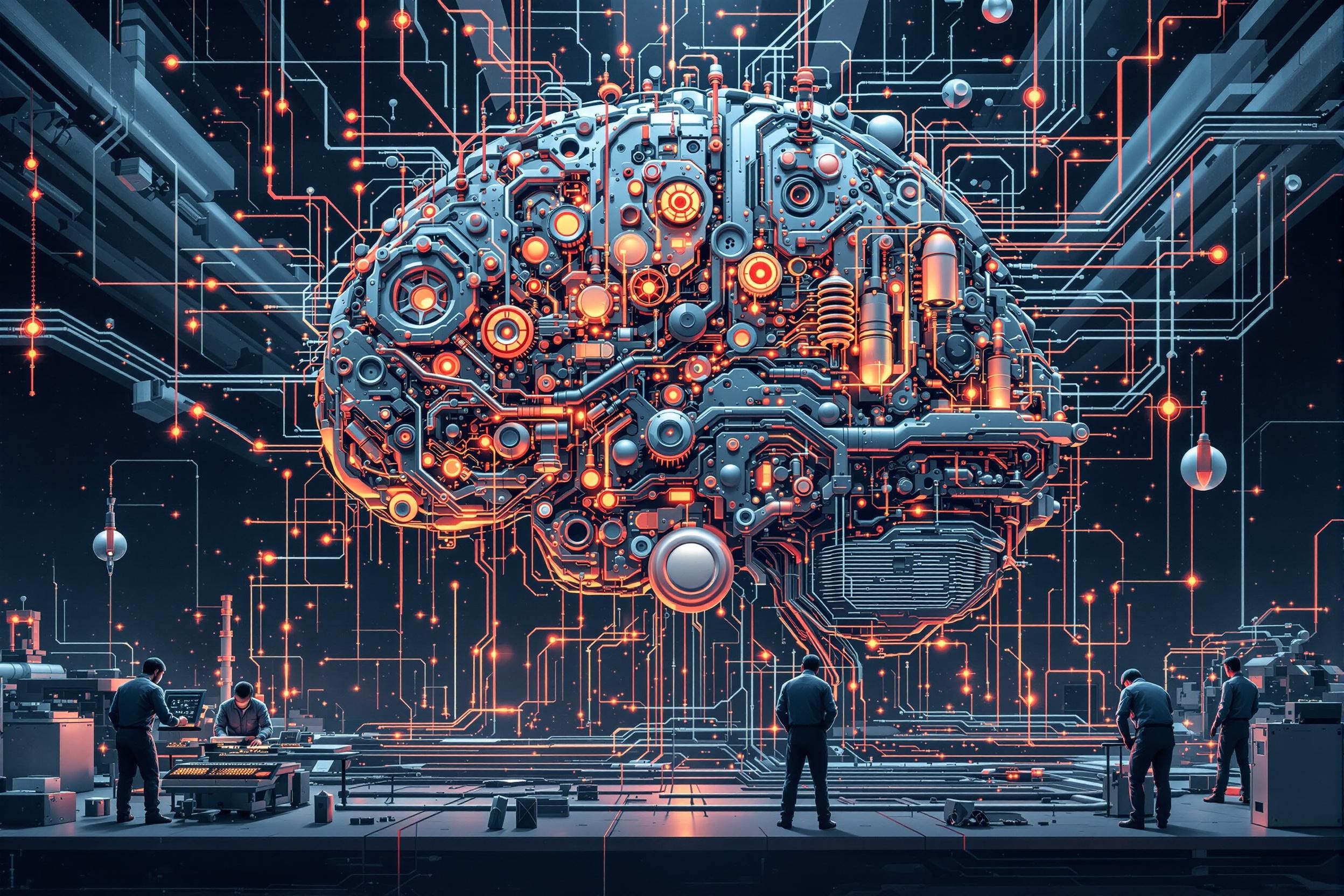